In this article, we will learn how to print the Pascal’s Triangle in Java, using a two dimensional (2D) array.
Well, first of all, let us understand what is Pascal’s Triangle and how is it formed.
Table of Contents
What is Pascal’s Triangle And How is it Formed
We can say that in Pascal’s triangle, each element is the sum of the two elements that lie directly above it (except the two slanting vertical boundaries/sides, which are always 1).
Now let’s visualize a Pascal’s Triangle of 5 steps
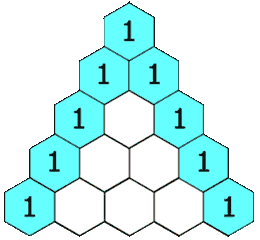
You May Learn more about Pascal’s Triangle on Wikipedia.
Now I will show you two different ways to print Pascal’s triangle in Java using a 2D array, up to N steps. (N is the value inputted by the user).
Java Program
Method 1
Simple Pascal’s triangle with no spacings.
In this method, we will only print Pascal’s triangle in the form of a right-angled triangle.
Scroll down more for the other style.
e.g. where n=5,
the output will be:-
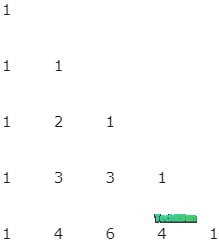
PROGRAM:
Note: I have used the Scanner class to take input from the user. BufferedReader and InputStreamReader can also be used.
import java.util.Scanner;
class Pascal_Triangle
{//opening of class
public static void main(String args[])
{//opening of main
Scanner sc=new Scanner(System.in);
int n,i,j,a[][];
//taking user's input.
System.out.println("HOW MANY STEPS?");
n=sc.nextInt();
a=new int[n][n];
//filling the 2D matrix.
for(i=0;i<n;i++){
for(j=0;j<=i;j++)
if(j==0 || j==i)
a[i][j]=1;
else
a[i][j]=a[i-1][j-1]+a[i-1][j];
}
//displaying the Pascal's Triangle as the output.
System.out.println("\nOUTPUT:\n");
for(i=0;i<n;i++)
{
for(j=0;j<=i;j++)
System.out.print(a[i][j]+"\t");
System.out.println();
}
}//clossing of main
}//closing of class
Method 2
Pascal’s Triangle with proper spacings
In this method, we will print Pascal’s triangle with proper spacings.
e.g. where n=5,
the output will be:-
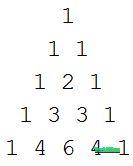
PROGRAM:
Note: I have used the Scanner class to take input from the user. BufferedReader and InputStreamReader can also be used.
import java.util.Scanner;
class Pascal_Triangle
{//opening of class
public static void main(String args[])
{//opening of main
Scanner sc=new Scanner(System.in);
int n,i,j,a[][],s;
//taking user's input.
System.out.println("HOW MANY STEPS?");
n=sc.nextInt();
s=n-1;
a=new int[n][n];
//filling the 2D matrix.
for(i=0;i<n;i++){
for(j=0;j<=i;j++)
if(j==0 || j==i)
a[i][j]=1;
else
a[i][j]=a[i-1][j-1]+a[i-1][j];
}
//displaying the Pascal's Triangle as the output.
System.out.println("\nOUTPUT:\n");
for(i=0;i<n;i++)
{
for(j=0;j<=s;j++)
System.out.print(" ");//printing blank spaces at the beginning of rows
s--;
for(j=0;j<=i;j++)
System.out.print(a[i][j]+" ");
System.out.println();
}
}//clossing of main
}//closing of class
Explanation
Let’s say the user wants the program to display 3 steps of the pascal’s triangle.
Then, N=3.
Then we declare an array containing 3 rows and 3 columns, that is, a 3×3 double dimensional array as shown below:
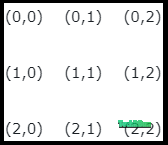
Now, the for i loop will run from 0 to less than 3 (i.e. 2), and the for j loop will run inside the i loop from 0 to i.
Here’s the dry run:-
Condition: if(j==0 || j==i) then a[i][j]=1 else a[i][j]=a[i-1][j-1]+a[i-1][j]
External Iteration (i) | Internal Iteration (j) | j==0 || j==i? | a[i][j] |
0 | 0 | true | a[0][0]=1 |
1 | 0 | true | a[1][0]=1 |
1 | true | a[1][1]=1 | |
2 | 0 | true | a[2][0]=1 |
1 | false | a[2][1]=a[1][0]+a[1][1]=2 | |
2 | true | a[2][2]=1 |
Now, if we fill the 2D Matrix with the values of a[i][j], we get:-

Looking closely, we can figure out this matrix is the right angled Pascal’s Triangle ignoring the zeros that are present above the diagonals.
Now, the Pascal’s Triangle can easily be printed using a nested loop, as shown in the programs above.
Variable Description
For Method 1 (Right Angled)
VARIABLE NAME | DATA TYPE | PURPOSE |
n | int | To accept the value of N from the user. N is the number of steps of the Pascal’s Triangle. |
i | int | Control variable of outer for-loop |
j | int | Control variable of inner for-loop |
a[][] | int | Double dimensional integer array to store the elements constituting the output Pascal’s Triangle. |
For Method 2 (With Proper Spacing)
VARIABLE NAME | DATA TYPE | PURPOSE |
n | int | To accept the value of N from the user. N is the number of steps of the Pascal’s Triangle. |
i | int | Control variable of outer for-loop |
j | int | Control variable of inner for-loop |
a[][] | int | Double dimensional integer array to store the elements constituting the output Pascal’s Triangle. |
s | int | To keep a count on the number of spaces printed at the beginning of each row. |
Algorithm
Note: If you are using method 1, please ignore the lines/characters in red.
- START.
- INCLUDE THE Scanner class FROM THE util package OF JAVA.
- DEFINE class Pascal_Triangle.
- DEFINE THE main FUNCTION.
- INSTANTIATE AN OBJECT “sc” OF THE Scanner class WITH (System.in) AS THE PARAMETER.
- DECLARE INTEGER VARIABLES (n,i,j,a[][],s).
- ASK THE USER TO ENTER THE VALUE OF N.
- STORE THE USER’S INPUT IN THE VARIABLE n.
- SET s=n-1.
- SET a=new int[n][n].
- REPEAT for i=0 to n (i<n)
11.1. REPEAT for j=0 to i (j<=i)
11.1.1. CHECK IF J IS 0 OR J IS 1. THEN, set a[i][j]=1. ELSE set a[i][j]=a[i-1][j-1]+a[i-1][j].
11.2. END OF for j - END OF for i
- DISPLAY “OUTPUT:”.
- REPEAT for i=0 to n (i<n)
14.1. REPEAT for j=0 to s
14.1.1. DISPLAY A BLANK SPACE. LET THE CONTROL BE ON THE SAME LINE.
14.2. END OF for j.
14.3. DECREASE THE VALUE OF s BY 1.
14.4. REPEAT for j=0 to i (j<=i)
14.4.1. DISPLAY a[i][j] AND A BLANK SPACE. LET THE CONTROL BE ON THE SAME LINE.
14.5. END OF for j.
14.6. DISPLAY AN EMPTY NEW LINE. - END OF for i.
- END OF THE main FUNCTION.
- END OF THE class.
- END.
If you have any further doubts or face any problem writing the program to display the Pascal’s Triangle using a 2D (two-dimensional) array, feel free to comment down below. I will try my best to help you out.
If you have any suggestions for other programs that I should solve and post here, do let me know in the comment section below.
If this article has helped you, do share it with your friends who might also be benefitted from this article.
Have a nice day!
Awesome Explanation Keep it up !!!!!!!!!
Thanks for your comment, Rasik!
Explained very well. Helped me a lot, thank you.
Awesome! Keep Coding!
Why is it not working for values greater than 5? Greetings
Hello!
It should work perfectly fine for all positive values. Below are the screenshots when run for 6 steps.
Method 1:
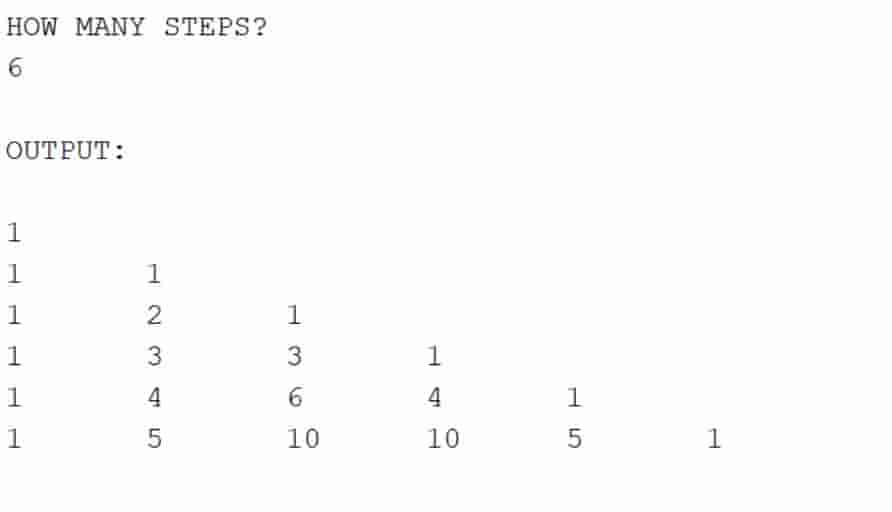
Method 2:
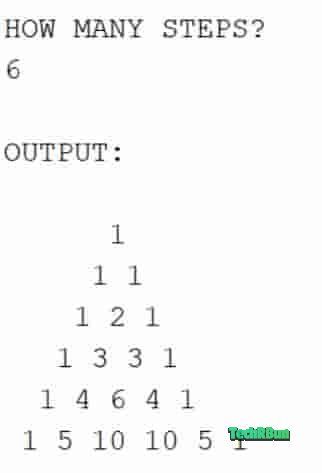
Amazing explanation. Really appreciate your efforts and dedication bro.