With the integration of technology into the retail industry, eCommerce platforms such as WooCommerce have seen rapid growth and usage. The open-source platform extends WordPress, a renowned content-management system, to include eCommerce functionalities, enabling users to set up their own online stores.
Essential to making the most of these tools is understanding basic programming languages like PHP, which WooCommerce is built around. The following discourse will delve into key aspects such as understanding the structure and core functionalities of WooCommerce, getting abreast with PHP and its syntaxes, and parsing URLs in PHP, key to extracting product IDs from product hyperlinks.
Table of Contents
Understanding WooCommerce
Getting a basic understanding of WooCommerce
Understanding WooCommerce is essential to operating it programmatically. WooCommerce is an open-source eCommerce platform specifically designed for WordPress. So, it is essential to have a basic understanding of WordPress and its functionalities before diving into WooCommerce.
Details of WooCommerce structure and core functionalities
WooCommerce works around products, carts, and orders. Its structure is composed of product pages, a shopping cart page, and a checkout page, while the core functionality handles everything from managing products to handling cart operations, checking out, and managing orders.
Extracting Product IDs from Product Hyperlinks in WooCommerce
In order to extract a product id from a product hyperlink programmatically in WooCommerce, some understanding of PHP is required.
Here are steps to do it:
Check the Product URL
Check the URL of the product for which you want to find the ID. The URL usually looks like https://yourwebsite.com/product/product-name
.
Create a PHP Function
To retrieve the product id from a product hyperlink, you’ll need to write a PHP function. Here’s an example:
<?php
function get_product_id_from_url( $url ) {
$path = parse_url($url, PHP_URL_PATH);
// Split the path into segments
$path_segments = explode('/', trim($path, '/'));
// Get the last path segment
if($path_segments){
$product_slug = end($path_segments);
// Get the product id from the slug
$product = get_page_by_path( $product_slug, OBJECT, 'product' );
return $product ? $product->ID : null;
}
return null;
}
?>
$product_id = get_product_id_from_url('https://yourwebsite.com/product/product-name');
Remember that you need access to your website’s code base to add these snippets. Always backup your website before making any changes to your code base and make sure you understand what the code is doing before adding it.
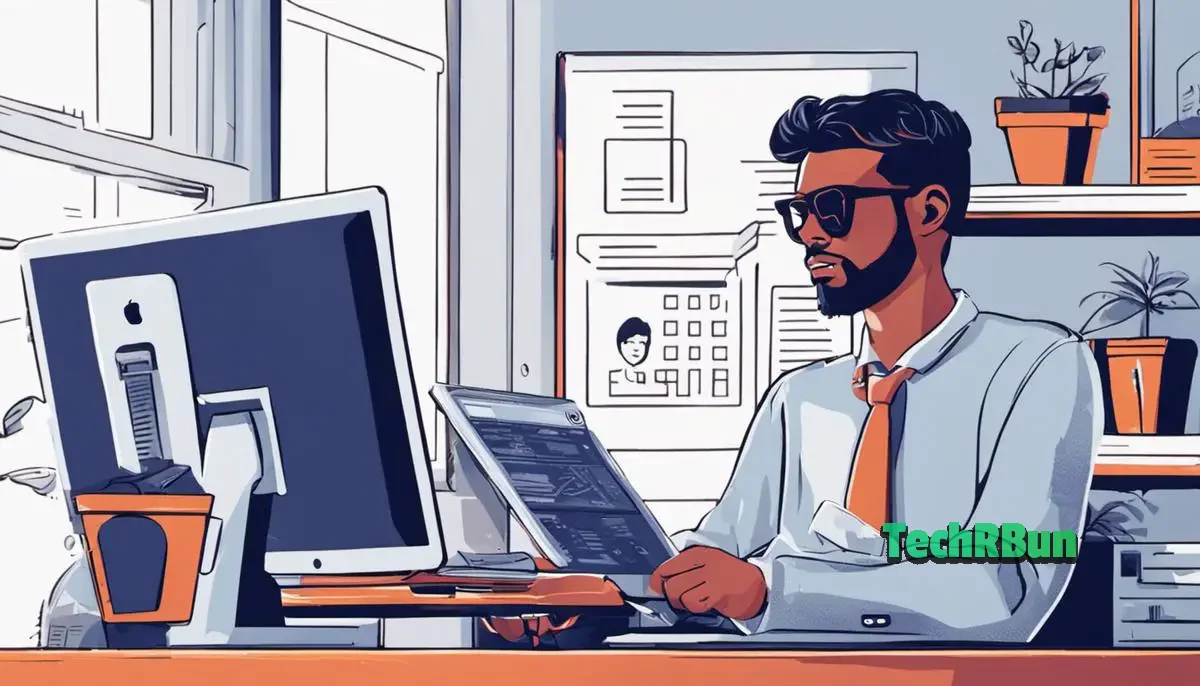
Learning To Modify WooCommerce With PHP
Understanding the Woocommerce Framework
Before moving further, it’s essential to understand the underlying framework of WooCommerce. WooCommerce is built using WordPress; thus, it heavily uses PHP. To work programmatically with the WooCommerce you need a sound knowledge of PHP and WordPress core functions.
Getting Familiar with PHP
In order to extract the product ID from a product link, you’ll first need to get comfortable with PHP, the programming language that WooCommerce is built in. Brush up on PHP syntax and basic functions as these will come in handy. Spend time learning about variables, strings, arrays, loops, and conditional statements in PHP. A strong grasp of these concepts is one of the keys to successfully extracting the product ID programmatically.
Cultivating an Understanding of WooCommerce URLs
Products in WooCommerce have unique URLs, usually structured like this: https://yourwebsite.com/product/product-name
.
The segment product-name is known as a ‘slug’ in WordPress terminology. Usually, it’s the sanitized version of the product name but it can be set manually from the product edit page.
Writing PHP Code to Extract Product ID
To extract the product ID from a product URL, we will use the combination of WordPress’s get_page_by_path()
and url_to_postid()
function. Below is a sample script that takes a product URL and returns the product’s ID:
ID;
} else {
return null;
}
} else {
return $post_id;
}
}
This will print the product id:
Explanation of the Code
In the PHP function get_id_by_slug
, ‘https://yourwebsite.com/product/product-name’ is the product URL from which we want to extract the Product ID.
We replace ‘https://yourwebsite.com/product/product-name’ with the URL of your product. The function first tries to get the post id from the URL using the WordPress function url_to_postid()
. If it fails, it tries to extract the slug from URL and then retrieves the complete Post data using the get_page_by_path
function and returns the ID of the post.
With this script in place, you now have a way to extract the Product ID from WooCommerce product URLs. Keep in mind that knowing PHP and server-side scripting concepts is a prerequisite to modify or extend this sample script further.
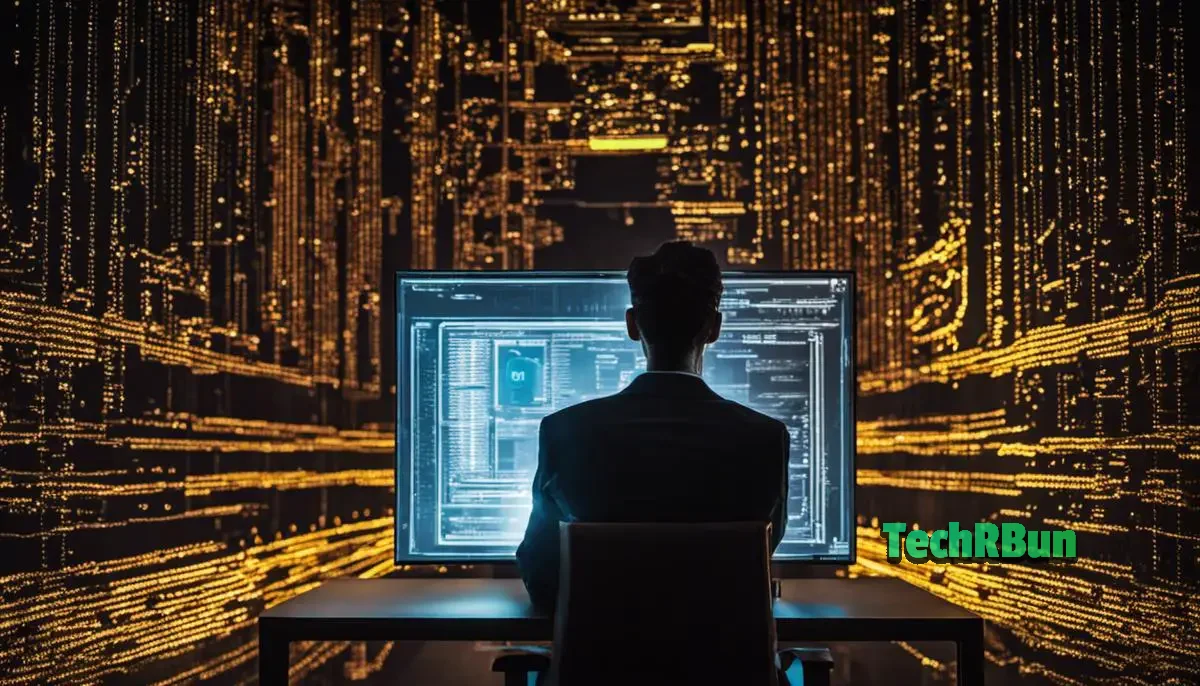
Working with URLs in PHP
Understanding URLs and Query Strings
In the digital world, Uniform Resource Locators (URLs) serve as roadmaps to specific web pages and resources. Each URL is a unique identifier for a piece of content on the web. Within these URLs, you might often notice different parts separated by characters like ‘?’ or ‘&’. This is known as a query string.
A query string is a part of a URL that carries particular parameters or data that servers use to deliver a specific page or information. For instance, in the URL ‘website.com/products?product_id=100’, ‘?product_id=100’ is the query string.
Working with URLs in PHP
PHP, as a backend language, provides easy ways to fetch and manipulate URLs. To get the current URL in PHP, we use the ‘$_SERVER’ superglobal array. Here’s how you would fetch the current URL:
$url = "http://".$_SERVER['HTTP_HOST'].$_SERVER['REQUEST_URI'];
echo $url;
Extracting Query String from the URL
Once you have the URL, you can extract the query string. PHP provides a function ‘parse_url()’ which is used for parsing URLs and returns an associative array containing any of the various components of the URL. The ‘PHP_URL_QUERY’ component of ‘parse_url()’ can be used to fetch the query string.
$query_string = parse_url($url, PHP_URL_QUERY);
echo $query_string;
Parsing Query Strings to Get Specific Data
Once we have the query string from the URL, we need to parse it to extract specific data. For example, product ID. PHP provides a function called ‘parse_str()’ that parses a query string into variables. Here’s how you can use it to get the product ID:
parse_str($query_string, $query_array);
$product_id = $query_array['product_id'];
echo $product_id;
Extracting Product ID from WooCommerce product hyperlink
The above described PHP URL parsing methods can also be used to extract the product ID from a WooCommerce product’s hyperlink. Consider a typical WooCommerce product URL : www.yoursite.com/product/sample-product/?id=20. You can extract the product ID from the URL using ‘parse_url()’ and ‘parse_str()’ methods as shown above.
$url = "www.yoursite.com/product/sample-product/?id=20";
$query_string = parse_url($url, PHP_URL_QUERY);
parse_str($query_string, $query_array);
$product_id = $query_array['id'];
echo $product_id; // Outputs '20'
The above code will output ’20’, which is the product ID.
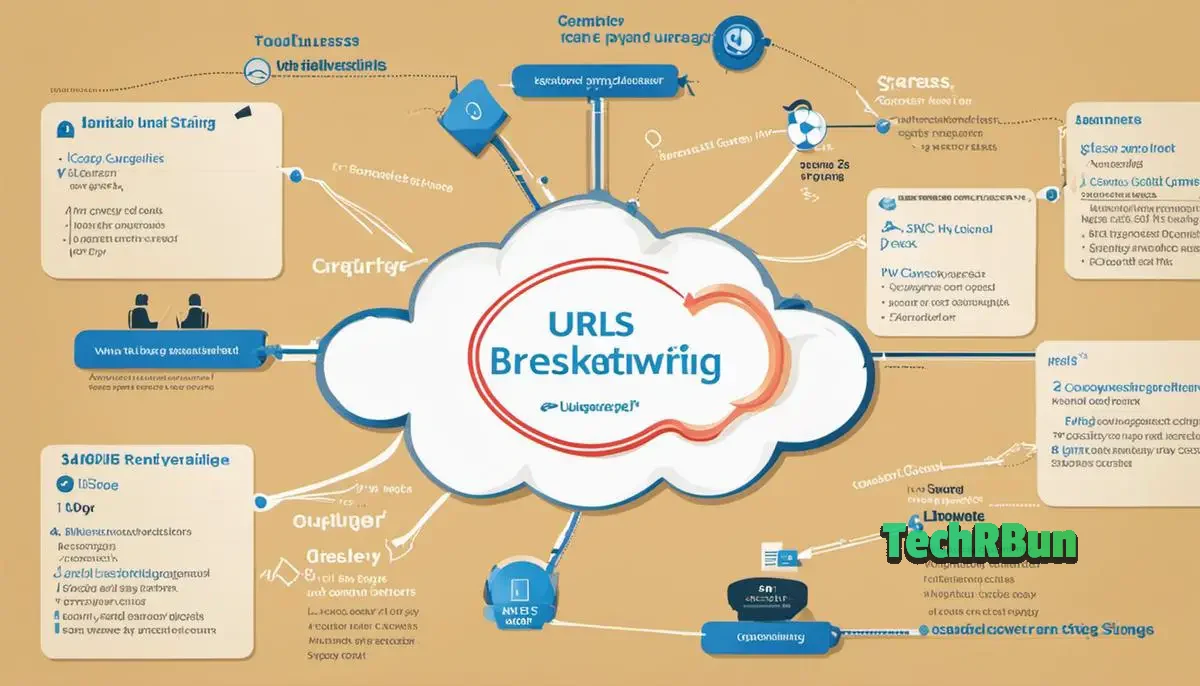
Practicing with WooCommerce PHP functions
Understanding PHP and URL Handling
To retrieve the product ID using a product’s hyperlink in WooCommerce, you will first have to fully comprehend PHP and URL handling. PHP, or Hypertext Preprocessor, is a scripting language while URL handling is the process of managing the URLs or hyperlinks of website pages. Both are instrumental to extract data from site links.
Familiarity with WooCommerce PHP Functions
Once you’re comfortable with PHP and URL handling, your next focus should be learning about WooCommerce PHP functions. WooCommerce is an open-source, completely customizable eCommerce platform for entrepreneurs worldwide, and it’s built on WordPress, the software that runs 26% of the web. WooCommerce PHP functions thereby refer to the PHP functionalities that allow fetching of data and performance of other actions.
Extracting Product ID from URL
One particular function you need to understand in WooCommerce is getting a product ID from a product hyperlink. Each hyperlink in WooCommerce contains the product ID. This ID is used to refer to a particular product, thus making it easier to handle product data in a systematic way.
A function to get a product ID from a URL could look something like this:
function get_product_id_by_url($link) {
$id = url_to_postid($link);
if ($id) {
return $id;
} else {
return false;
}
}
In this example, the function ‘url_to_postid()’ is used to capture the product ID from a hyperlink. This function, provided by WordPress, gets the ID of a post or page by its URL.
Grasping the Structure of Product Hyperlink
Understanding the structure of a product hyperlink is equally important. A typical product hyperlink in WooCommerce seems like this:
“https://example.com/product/product-name/”. Here, ‘example.com’ is the domain, ‘product’ is the product post type, and ‘product-name’ is the WooCommerce product slug and most often also the product name. The product ID is thus embedded in the hyperlink architecture and can be programmatically extracted using the function we discussed above.
By practicing WooCommerce PHP functions and understanding the structure of a WooCommerce product hyperlink, you can effectively retrieve the product ID using a product hyperlink. Remember, the more you practice, the better you get at coding!
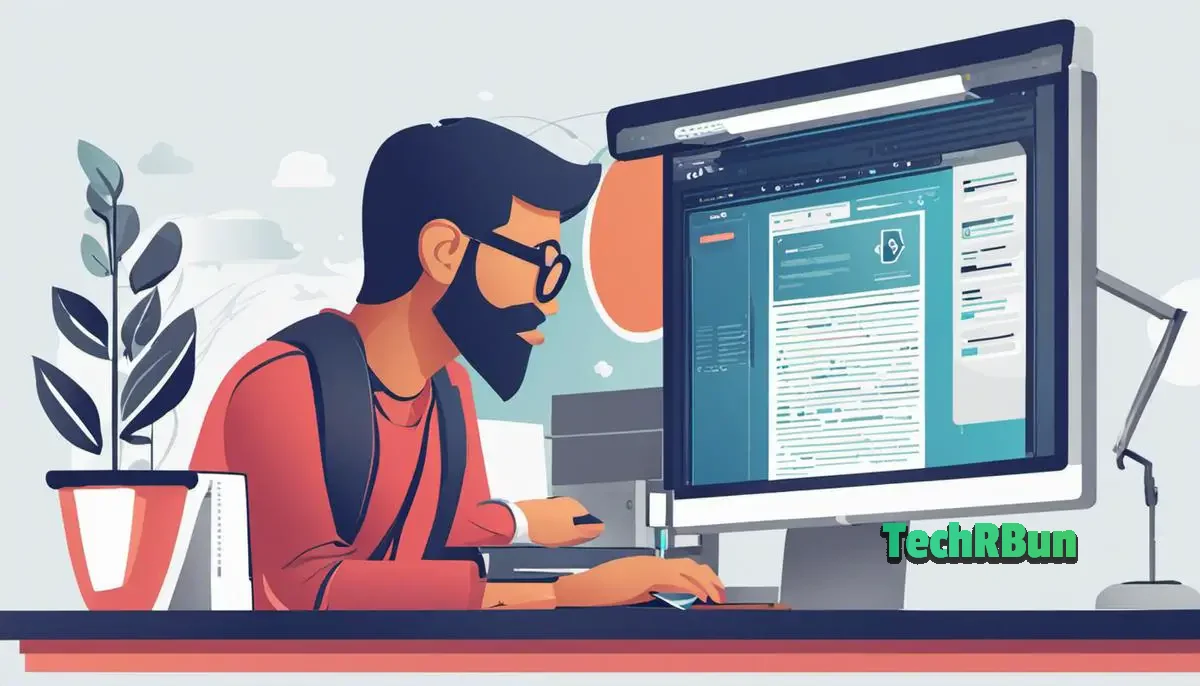
Armed with knowledge on PHP and how URL handling is achieved, you are set to conquer the mechanics of WooCommerce. It’s all about practice and more practice, especially with the WooCommerce PHP functions.
Understanding these functions will enable you to identify product ID from a URL and will provide you with insights into the overall structure of a product hyperlink. Therefore, embedding programmatically inclined skills in WooCommerce is not only useful but also a necessary tool in the development and management of a functional and comprehensive online store. Your journey towards mastery starts now.