![Why JavaScript Does Not Support Function Overloading? [In-depth Analysis And Explanation]](https://www.techrbun.com/wp-content/uploads/2023/09/Why-JavaScript-Has-No-Support-For-Function-Overloading-1-1024x576.png)
Breaking down the complex world of programming is no easy task but it yields fascinating revelations on how different languages operate.
This discussion ventures into a critical comparison: function overloading and its workings across programming environments, particularly its absence in JavaScript.
Understanding function overloading sets the groundwork for approaches in languages like C++ or Java, displaying how unique blocks of code can be assigned similar names yet deliver different outcomes based on input parameters.
The following discussion delves deeper into why JavaScript inherently lacks function/method overloading, owing to its distinct interpretation of functions.
Table of Contents
Understanding Function Overloading And Its Absence In JavaScript
Function Overloading: A Brief Overview
Function overloading is a feature of certain programming languages that allows the creation of several functions with the same name but different parameters.
These versions of a function will perform different tasks, depending on their parameter lists.
The decision of which function version to execute is made at compile time, based on the number and type of arguments.
Languages such as C++ and Java feature function overloading, which can simplify code and improve readability.
Understanding Function Overloading through C++ and Java
To understand function overloading better, here are a few examples from C++ and Java.
In C++, it’s possible to create multiple functions with the same name but different parameters.
For instance, if we have three functions named “add” – one which adds two integers, another which adds two doubles, and a third that concatenates two strings – each “add” function will perform a different action, depending on whether it receives integers, doubles, or strings as arguments.
In Java, function overloading is accomplished similarly, but with a few key differences.
First, Java determines the version of an overloaded method to use based on the compile-time type of the reference, not the run-time type of the object.
Second, like C++, Java uses the number, order, and type of arguments to determine which version of an overloaded method to use.
This pre-runtime selection of functions adds an element of predictability and control to coding.
Reason Behind JavaScript’s Non-Support of Function Overloading
Although function overloading is a common feature in many programming languages, it is not supported in JavaScript.
This is largely due to JavaScript being a dynamically typed language.
In other words, JavaScript only decides on the types, values, and function calls during the program’s execution or runtime, not while the code is being compiled.
As such, when function overloading is attempted in JavaScript, the interpreter does not hold the function signature into account – meaning it overlooks the number, type, or order of parameters, causing overloading to be ineffective.
Furthermore, JavaScript is designed in such a way that if there are multiple definitions of functions carrying the same name, the last defined function in the code will overwrite all prior definitions.
This indicates that even when you craft multiple functions with the same name, differing parameters, and operations, only the last function will be recognized and implemented.
The issue therefore isn’t that JavaScript lacks the capability to handle complexity, but rather that its design and mechanics do not accommodate function overloading, unlike legacy statically typed languages.
Ultimately, it wouldn’t be incorrect to state that JavaScript doesn’t support function overloading, in the traditional sense.
Instead, its dynamic nature interprets this means differently – functionality is determined by the last function declared with that specific name.
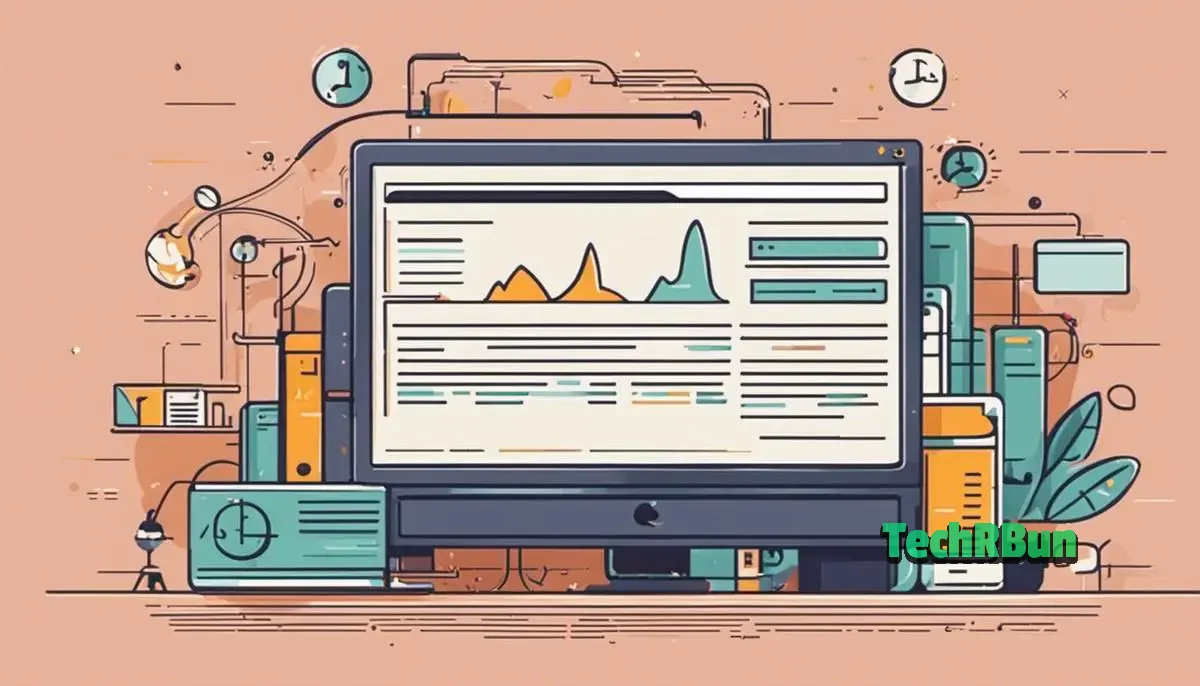
Understanding JavaScript Functioning And Method Overloading
Getting to Grips with Functions in JavaScript
As a dynamic and loosely typed language, JavaScript exhibits unique attributes that set it apart from other programming languages such as Java and C++.
A central feature that demonstrates this uniqueness is the role of functions in JavaScript.
This characteristic is not only pivotal to the overall structure of JavaScript, but also differentiates it from its statically-typed counterparts.
The Concept of Overloading in JavaScript
Function overloading, a feature found in statically-typed languages such as Java or C++, refers to the ability of a single function to cater to different types and combinations of data parameters.
For example, a function named calculate could perform addition with two integer parameters, yet still execute a different block of code with two string parameters.
In JavaScript, however, function overloading falls outside of its design.
JavaScript functions do not consider the number, sequence, or type of arguments they receive when executed.
This occurs because, during interpretation, JavaScript replaces a function if a new one with the same name is declared. Ultimately, only the most-recent version of the function remains feasible for execution.
Attempts at “Overloading” in JavaScript
While traditional overloading does not exist in JavaScript, developers can approach overloading through various workarounds.
Different functions can be executed depending on the length or data type of the supplied argument. Developers also often utilize optional parameters or objects with specific properties to extend a function’s capabilities.
Nevertheless, it’s important to remember that these are merely workarounds. They are pseudo-overloading efforts that do not involve genuine overloading in the way other languages implement them.
Prototyping and JavaScript
Prototyping in JavaScript further illustrates why overloading doesn’t exist. Prototyping allows an object to inherit properties and methods from another object.
This mechanism is used to simulate classic object-oriented features such as classes, yet still doesn’t allow for function overloading. Regardless of prototyping, a function will still replace any previous function with the same name.
Taking A Deeper Dive Onto Why Function Overloading Doesn’t Work in JavaScript
The Structure JavaScript Methods
In JavaScript, functions are treated as first-class objects.
When you define a function, JavaScript does not associate the function’s body with its name and parameters.
Instead, it creates a function object and assigns it to a variable with the name of the function.
In other languages, if you define two functions with the same name but different parameters, the compiler can distinguish between them based on their parameter lists.
But in JavaScript, defining a function with the same name essentially reassigns the variable to a new function object, losing the reference to the original function.
For example, look at the following code:
function add(a, b) {
return a + b;
}
function add(a, b, c) {
return a + b + c;
}
In JavaScript, instead of having two separate add
functions you could call with two or three arguments, you would just have the latter add
function that expects three arguments.
The first add
function gets completely overwritten.
Exploring Additional Function Capabilities in JavaScript
While JavaScript may not traditionally support function overloading, there are several workarounds that emulate this feature.
One such method involves the arguments
object, an array-like object that stores the values of arguments passed to that function.
By evaluating the arguments.length
property, it’s possible to determine how many arguments were transferred and adjust the function accordingly.
Another strategy is to pass an object as a parameter.
The operation of the function can then be modified based on this object’s properties. In this way, distinct objects can be used to call forth differing behaviors.
However, it’s worth noting that these techniques, while embodying some aspects of function overloading, are not a true replacement for the feature itself.
The foundational reason JavaScript doesn’t accommodate function overloading centers on its treatment of functions as objects and its approach to variable assignment.
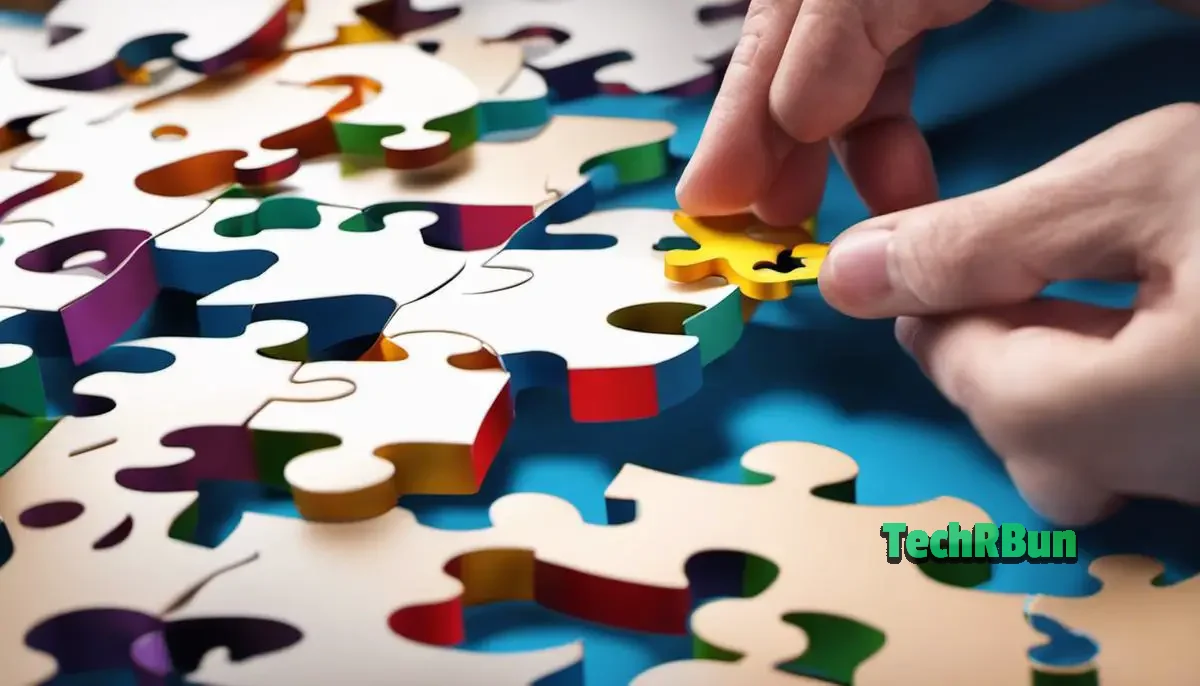
Alternatives to Function Overloading in Javascript
Decoding Function Overloading in JavaScript
Understanding that JavaScript does not natively support function overloading is a pivotal aspect of navigating the language.
Many programming languages allow for the definition of several functions bearing the same name but different parameters, each performing separate tasks.
The correct function would then be selected based on the passed arguments at runtime – a feature known as function overloading.
However, this built-in feature is absent in JavaScript. When defining multiple functions with identical names in JavaScript, the most recently defined function overpowers the preceding ones, and only the final function will be executed.
This is predicated on the fact that functions in JavaScript amount to variable references to functions. Consequently, redefining a function merely redirects the variable to a new function.
Emulating Function Overloading through Default Parameters
Although JavaScript does not directly support function overloading, there are several ways to more or less achieve the same functionality.
One method is to use default parameter values.
This lets you define a function with multiple parameters with default values that JavaScript automatically fills in if no value or undefined is passed for those parameters at function call.
Let’s look at an example:
function myFunction(x, y = 'default_y', z = 'default_z'){
console.log(x, y, z);
}
myFunction('input_x'); // Output: "input_x" "default_y" "default_z"
myFunction('input_x', 'input_y'); // Output: "input_x" "input_y" "default_z"
myFunction('input_x', 'input_y', 'input_z'); // Output: "input_x" "input_y" "input_z"
In this example, myFunction
appears to have different behaviors based on the number and type of arguments, emulating function overloading.
Using Optional Parameters
Another technique to emulate function overloading in JavaScript is by using optional parameters.
JavaScript allows function calls with any number of arguments, regardless of the number of parameters defined in the function.
When an argument is not passed for a specific parameter, its value is undefined.
Let’s look at an example:
function myFunction(x, y, z) {
if(z === undefined){
console.log(x, y);
} else {
console.log(x, y, z);
}
}
myFunction('input_x', 'input_y'); // Output: "input_x" "input_y"
myFunction('input_x', 'input_y', 'input_z'); // Output: "input_x" "input_y" "input_z"
Method Overloading
JavaScript also supports method overloading, a variant of function overloading, using object prototypes.
This works because JavaScript treats functions as first-class objects, and functions can be assigned as values to object properties.
So, you can define multiple methods of an object with the same name as properties of the object, and then use the appropriate method based on the number or type of the arguments.
Let’s look at an example:
var myObject = {
myMethod: function(x, y) {
if (y === undefined) {
console.log(x);
} else {
console.log(x, y);
}
}
};
myObject.myMethod('input_x'); // Output: "input_x"
myObject.myMethod('input_x', 'input_y'); // Output: "input_x", "input_y"
In this example, myMethod
in myObject
behaves differently based on the number of arguments, emulating method overloading.
In conclusion, while JavaScript doesn’t support function overloading in the traditional sense, there are several ways to emulate this behavior.
By carefully planning the architecture of the application, developers can effectively manage multiple functionalities using the same function or method name.

Despite Javascript’s quirks and its noticeable absence of function overloading, programmers have ingeniously crafted alternatives to ensure versatile software development stays within reach.
By mastering Javascript’s default parameter values, optional parameters, and method overloading, developers can emulate the behavior of function overloading up to a point, keeping multi-purpose programming alive.
While it may not offer the classic approach to function overloading, Javascript’s unique handling of functions opens doors to different, equally impressive, programming strategies that continue to move the field forward.
Summing it up, JavaScript, while it does not support traditional function overloading, offers several alternative methods that enhance the versatility of functions.
This is rooted in its design as a web scripting language – it’s dynamic and streamlined, yet comes with its own set of peculiarities.
The absence of function overloading should not be seen as a limitation, but rather, it’s an inherent characteristic of how JavaScript treats functions.